UI Goodies examples repository
https://github.com/bitfinexcom/bfx-stuff-ui : small examples to master Bitfinex UI Goodies
Chart Marks
Overview
Chart markers allow you to display certain events, such as important news, on the Bitfinex UI chart. All markers are associated with a specific timestamp which determines the candle above which the mark will be shown.
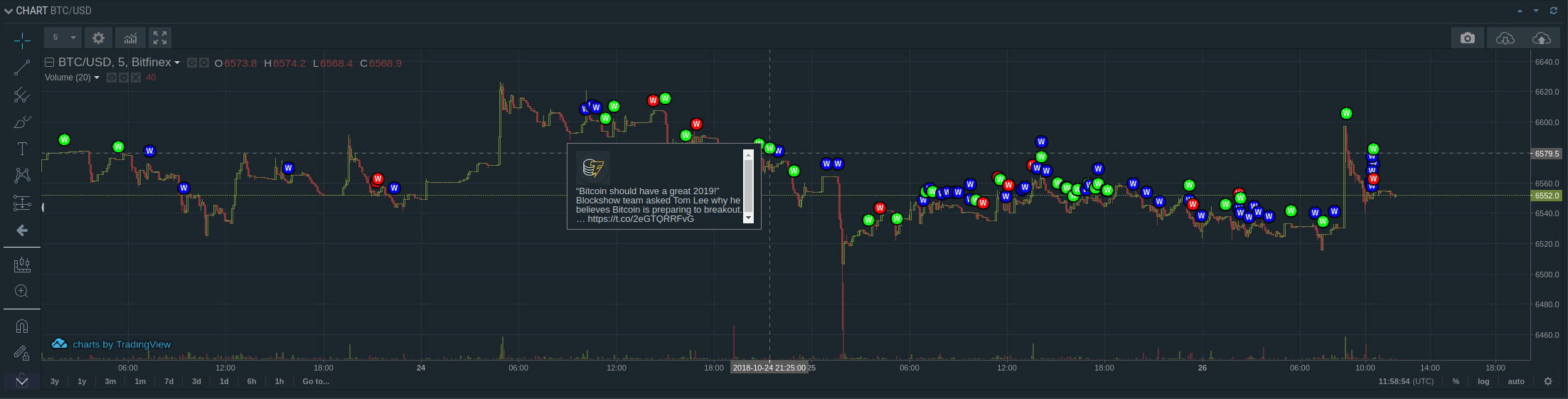
API
Chart markers can be manipulated with websocket messages:
Create a new mark
wss.send(JSON.stringify([0, 'n', 12345, {
type: 'ucm-ui-chart',
info: {
type: 'marker_create',
id: 'mark_0',
ts: Date.now(), // timestamp in milliseconds
symbol: 'tBTCUSD', // not required. If not present applies to all symbols
content: 'lorem <a href="https://www.bitfinex.com/assets/bfx-stacked.png">here</a>', // content to show in tooltip
color_bg: '#FF1133', // RGB
color_text: '#FF1133', // RGB
color_border: '#FF1133', // RGB
label: 'M', // marker letter
size_min: 8,
}
}]))
Clear all existing marks
wss.send(JSON.stringify([0, 'n', 12345, {
type: 'ucm-ui-chart', info: {
type: 'marker_clear',
} }]))
Fields
Name | Type | Required | Description |
---|---|---|---|
type | String, one of [‘marker_create’, ‘marker_clear’] | yes | The type of the action |
id | string | yes | Unique id of the mark. It’s necessary to allow the UI to distinguish the marks, and to easily determine that the given mark is already added to the chart in a possible scenario in which the same mark is broadcasted multiple times. |
ts | number | yes | Unix timestamp in milliseconds of the event. The mark of the event will be associated with the candle that overlaps with this moment in time. |
symbol | string | no | Symbol that the mark applies to e.g. “tBTCUSD”. If not provided, or provided value is “ANY” the mark applies to each existing pair. |
content | string(HTML) | no | HTML content displayed in the tooltip. Tooltip appears on mark hover and disappears on mark blur. This library is used to sanitize HTML to prevent potentially malicious tags from being injected: https://www.npmjs.com/package/sanitize-html |
color_bg | string | no | Background color of the mark. Must be in a form of RGB color such as “#FF0099” |
color_border | string | no | Border color of the mark. Must be in a form of RGB color such as “#FF0099” |
color_text | string | no | Text color of the mark. Must be in a form of RGB color such as “#FF0099”. Should not be confused with tooltip text color. (See “label” below) |
label | string with length = 1 | no | Optional single char that is displayed inside the mark circle when it’s zoomed in enough. Note: chart marks tend to become smaller when zooming out, and become bigger when zooming in. See also “size_min”. |
size_min | number | no | Minimum mark size in pixels. See also “label” above. |
HTML Sanitize settings
Definition of allowed HTML tags and attributes
const SANITIZE_SETTINGS = {
allowedTags: ['h3', 'h4', 'h5', 'h6', 'blockquote', 'p', 'a', 'ul', 'ol',
'li', 'b', 'i', 'strong', 'em', 'code', 'hr', 'br', 'div',
'table', 'thead', 'caption', 'tbody', 'tr', 'th', 'td', 'pre', 'img'],
allowedAttributes: {
a: ['href', 'name', 'target'],
img: ['src', 'height', 'width'],
},
}
Custom Notifications
Broadcasts a custom notification to all connected websockets belonging to the authenticated user.
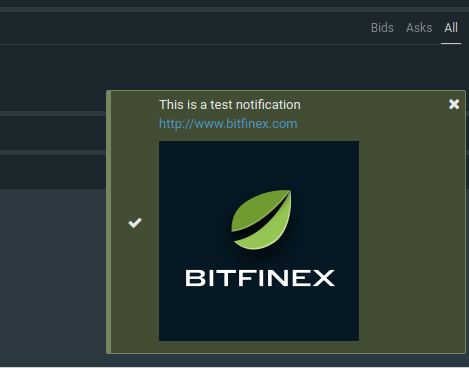
Simple notification
Fields
Name | Description |
---|---|
message | Notification content |
type | all, browser, desktop |
level | success, info, warning, error |
image | URL to any image (not supported for desktop notifications on macOS) |
link | URL to open when notification is clicked |
title | Header content |
ttl | Time in milliseconds to display notification for |
sound: { tone: TONE } | TONE=tone|wob|tripleChime| tripleBeep|doubleTap|pingUp| pingDown |
Functionality
- Display entire message content
- Play a sound, if provided, according to the specified user setting or provided tone (see structure above)
- Display an image, if provided, underneath the message content
- If a link is provided, the notification should open the link
- Display the title, if provided, above the message
- Close after either the provided TTL or the default
wss.send(JSON.stringify([0, 'n', 12345, {
type: 'ucm-notify-ui', info: {
type: 'all',
level: 'success',
image: 'https://www.bitfinex.com/assets/bfx-stacked.png',
link: 'http://www.bitfinex.com',
message: 'This is a test notification',
sound: {
tone: 'pingUp',
}
} }]))
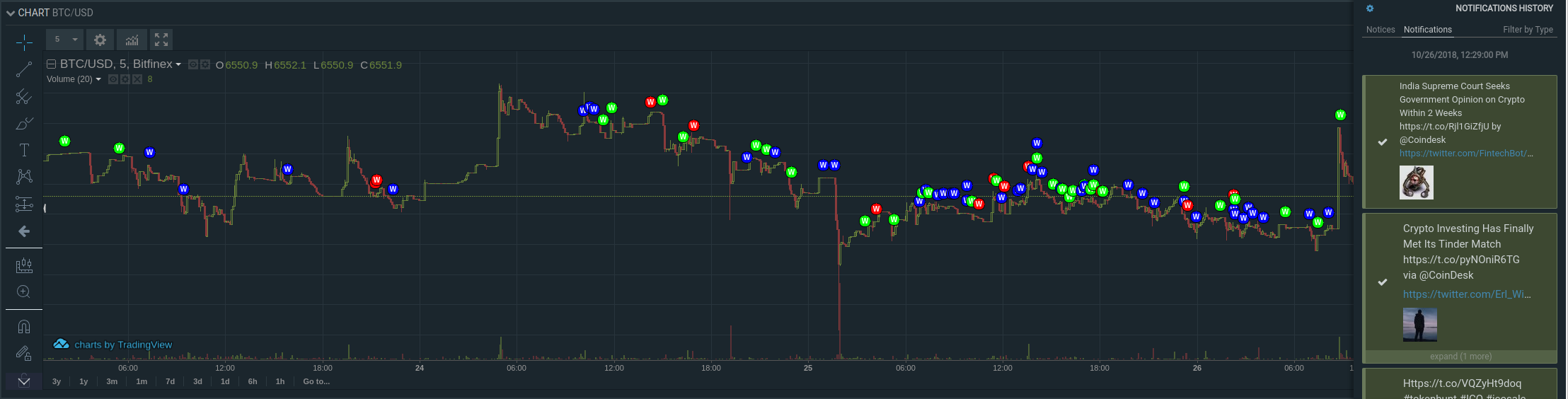
Chart Markers + Custom notifications from Twitter